Posts Tagged PHP
Poking holes in PHP object privacy
Posted by Howard Yeend in PHP on November 1, 2010
PHP provides a decent model of class member visibility, with public, private, and protected members to help you define tight APIs for your objects and show other developers how your object is supposed to be used. But used naively, PHP’s ‘magic methods’ can easily and subtly subvert this system, making everything public.
If you’re still new to object oriented programming in PHP5, think of “public” as roughly analogous to “my function’s arguments” and “private” as “local variables inside the function”. You wouldn’t want someone calling your function and messing with the local vars, and you wouldn’t want someone using your object messing with its private members.
Magic methods provide functionality like catching references to methods and properties which are not visible to us, and doing special things with them. Magic methods have always struck me as a bit weird, and whenever you bring them up in discussions online, there’s always a few people with reservations about them – efficiency, clarity, use-cases and so on.
I’m still in two minds; they can be useful in some circumstances, but here’s one reason why they could be considered harmful: Used carelessly, they can easily enable an OOP antipattern where all class members become public, even those declared as private or protected in the class definition. Read the rest of this entry »
Overloading in PHP
Posted by Howard Yeend in PHP on October 10, 2010
Murray Picton wrote up a blog post today on overloading functions in PHP. Overloading is a useful feature of many languages. Murray gives a nice definition in his post:
Overloading a function is the ability to define a function more than once with a different set of parameters for each one and then when it is called, the version of the function that matches the parameter set will be executed.
so you can define function foo(String $a) and foo(Array $a) and a different version will be called depending on how you call it. But, PHP’s idea of overloading is completely different and not really related.
Hello ladies, look at PHP, now back to Java, now back to PHP. Sadly, PHP’s not Java, but it can look (a bit) like Java if you stop using lady-scented functions and start using method overloading.
I just wanted to get that quote in somehow.
Murray’s solution is to use func_get_args inside your function, and perform some logic to switch execution to a different branch depending on what we find there. Something like:
function foo() {
$args = func_get_args();
if(is_string($args)) { /* do some stuff */ }
else if(is_array($args)) { /* do some stuff */ }
}
This works well enough, but there are a few things I don’t like about it:
- You have to put all the code into one function, instead of literally overloading multiple functions
- The “which version am I” code and the actual functionality are intermixed inside the function
I thought an OO solution might mitigate against these things, allowing us to write completely separate functions, but which can be called with the same name, a different one being executed depending on the arguments. I’m not certain my solution is an improvement over Murray’s, but it’s a different approach if nothing else:
Fast PHP array_unique for removing duplicates
Posted by Howard Yeend in PHP on June 21, 2010
PHP’s native dedupe function, array_unique, can be slow for large amount of input. Here I’m going to talk about a simple function that performs the same task but which runs a lot faster.
Often, people spout PHP optimisation advice that is incredibly misguided, so I want to make it clear up-front that you should be benchmarking your scripts using xdebug before you start optimising, and very often the real bottlenecks cannot be avoided by using “micro-optimization”. Here’s a nice PDF on Common Optimization Mistakes.
Now I’ve got that disclaimer out of the way, I believe it’s OK to talk about making a faster array_unique because it’s often used on large amounts of data – for example removing duplicate email addresses, and as such there are genuine use cases for a fast array_unique in PHP. Also our fast_unique function can be several seconds faster than array_unique so we’re not necessarily talking about micro-optimisation here; on 200k duplicate entries, array_unique takes nearly 5 seconds on my windows dev box, fast_unique takes <1 second on the same data. On 2 million entires the results are staggering – performance graphs are given below (but then, why are you doing that kind of work in PHP?).
The function looks like this:
Read the rest of this entry »
Fast PHP – effective optimisation and bottleneck detection
Posted by Howard Yeend in PHP, Productivity, web on April 18, 2010
PHP is not the fastest language on earth. That honour probably goes to machine code. But like many high-level languages, PHP provides some handy abstractions, like named variables, hashmaps (associative arrays), a C-like syntax, object oriented capabilities, loose typing and so on – we trade processing speed for development ease.
So it’s quite a common problem that people find their large PHP web applications running quite slowly.
Here are some frequently encountered bottlenecks found in web applications generally, and PHP specifically:
Super Useful Web Dev Tools
Posted by Howard Yeend in Firefox, javascript, PHP, Productivity, Programs on March 17, 2010
OMG, it’s been a whole month since my last update.
I have draft posts about all kinds of Good Stuffâ„¢, but none are quite publishable yet. So today I’m just going to point you at a few great resources I use all the time while doing my web development magic:
allRGB Entry – PHP Image Manipulation
Posted by Howard Yeend in PHP on February 10, 2010
The objective of allRGB is simple: To create images with one pixel for every rgb-color (16777216 to be exact); not one color missing, and not one color twice.
What a cool project! As regular readers will know, I love messing about with image manipulation in PHP, so when I heard about the allRGB project I knew I had to make an entry for it. A few false starts and about half an hour later, I proudly submitted my first entry, a 4096×4096 PNG image containing every single possible RGB colour. As one redditor put it, “It’s like poetry, just without words.”
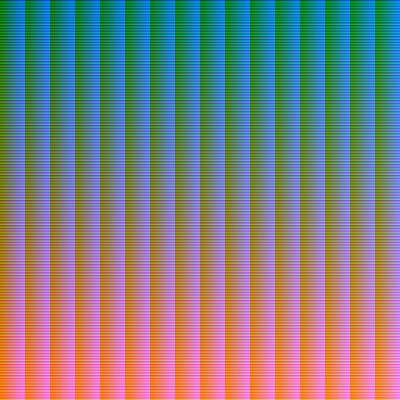
Click for the high resolution (only 173Kb)
And now on to the code:
Read the rest of this entry »
Textpad PHP manual lookup tool
Posted by Howard Yeend in PHP, Productivity on February 1, 2010
A little tip for those of us using textpad to develop in PHP. How often do you find yourself having to go back to PHP.net to check up on a function – is it ($needle, $haystack) or ($haystack, $needle)? I can never remember! With this tool I just need to highlight the function in textpad, press Ctrl-1 and up pops php.net in a new tab, opened on that function’s manual entry. Neat huh?
Here’s how:
Howard Yeend: UK Web Developer CV
Posted by Howard Yeend in (misc), PHP on August 1, 2009
Northampton UK /
Availability
Not currently seeking work. Sorry! (updated Mar 2013)
PHP error handling
Posted by Howard Yeend in PHP on July 5, 2009
Does this type of error handling code look familiar?
function doFunction($var) {
if(is_numeric($var)) {
/* do some stuff*/
} else {
return -1;
}
}
BLEH. How ugly is that? There’s no indication whether -1 is actually an error or a valid return value, or what it means. And other functions might use false to indicate errors so there’s inconsistency. So I’ve written a very simple function to help you give meaningful PHP error messages.
Read the rest of this entry »
PHP 5.3.0 Released!
Posted by Howard Yeend in PHP on July 1, 2009
If you’re still stuck in the old PHP 4 days, please please please take today’s release of PHP 5.3.0 as your cue to start learning about the wonderful world of object oriented PHP 5.
5.3.0 isn’t a hugely interesting release, but getting your PHP4 code compatible with 5 will ease the process when PHP 6 comes along. PHP6 is really where it’s at:
What’s out:
No more register_globals (finally)
No more magic_quotes (you kinda liked magic quotes? Admittedly it was handy, but when you think about it, having code that may or may not be sanitised depending on a php.ini setting is a Bad Ideaâ„¢)
No more HTTP_GET_VARS and cousins. Just change to $_GET etc and you’re fine.
What’s in:
A bunch of minor fixes aaand:
Namespaces – so we can section off bits of code properly. Woohoo! more info here.
Short post today I know, but I’ve got some real coding to do :) If you’re not doing anything better, go download PHP and have a play with it.
Recent Comments